The greatest concern faced by UI developers is the performance issues in situations where functions are being executed excessively and in some cases unnecessarily
There are two ways in order to control the execution of functions using Debounce and Throttle, which come in handy when on handling events such as mouse scroll or window resizing.
A simple explanation of debounce and throttle is that the number of times a function is executed over a period of time is throttle and the time passed between each execution of a function is implemented as debounce.
Real Time Example:
Debounce: The perfect example for debounce would be resizing of the window in a desktop. Imagine we are to get the value of the screen size, but it would be useless to execute the function as the user drags on the size of the screen but we would be interested only in the final value of the screen size after it has been resized, as explained in the link(https://css-tricks.com/debouncing-throttling-explained-examples/)
Throttle: An example of throttle can be explained through the infinite scroll, where we have to determine the scroll position and load data before the user reaches the bottom. In this case, it wouldn’t be advisable to use debounce, as it would allow us to call the function only after the user stops scrolling. But throttle would ensure that we constantly check how far is the user from the bottom and act accordingly.
The major advantage of throttle over debounce is that we have the option to store ignored executions and execute them at last after all the function calls are made
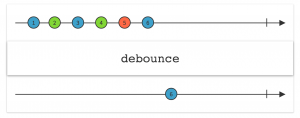
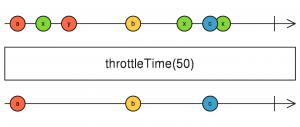
Implementation:
A code sample for implementation of debounce//original code from Jhey Tompkins blog //https://medium.com/@_jh3y/throttling-and-debouncing-in-javascript-b01cad5c8edf var debounce = function(func, delay) { var inDebounce = undefined; return function() { var context = this, args = arguments; clearTimeout(inDebounce); return inDebounce = setTimeout(function() { return func.apply(context, args); }, delay); } }The above function could be linked to a click event as follows:
debounceBtn.addEventListener(‘click’, debounce(function() { return console.log(‘Hey! It is’, new Date().toUTCString()); }, 3000));In the above code, when the button click event is called the function gets executed with the delay of 3 seconds, but if another click event is triggered before the execution of first click, then it is replaced by the second event. Similarly, a code implementation for throttle would be
//original code from comment of maninak //https://gist.github.com/makenova/7885923 function throttle(func, msWait) { var time = Date.now(); return function() { if ((time + (msWait || 100) – Date.now()) < 0) { func(); time = Date.now(); } } }Can be used as:
window.addEventListener(‘scroll’, throttle(myOnScrollFunction), false);The above code ignores execution of the function for the events that are being called within 100ms of execution of the previous function call.