Introduction:
Node.js is a powerful JavaScript platform based on Google Chrome’s JavaScript V8 Engine that is used to create I/O heavy web applications such as video streaming websites, online chat applications, single-page software applications, and other web application apps.
Both established, huge businesses and recent startups use Node.js API. The platform is utilized by hundreds of developers worldwide and is open-source and free. It has various benefits, making it frequently a better option than other server-side technologies like Java or PHP.
Web application:
As you may already be aware, a web application is a software design pattern that runs on a server and is displayed by a client browser, using the internet to access all of that software application’s resources. Typically, it can be divided into three parts:
- Client
- Server
- Database
Client:
A web application’s front end is where users interact. The front end is typically constructed using JavaScript-based frameworks like ReactJS and Angular, which aid in application control flow design, and languages like HTML and CSS.
Server:
The server accepts client requests, carries out necessary operations, and returns answers to the clients. It served as a middleware between the front end and stored data to allow client activities on the data. The most common technologies used to create and manage a web server are Node.js, PHP, and Java.
Database:
The data for a web application is kept in a database string connection. The Client can request the data’s creation, modification, and deletion at the exact time. Among the most widely used databases for storing data for web applications are MySQL and MongoDB.
Node.js server architecture:
Node.js manages several concurrent clients using the “Single Threaded Event Loop” hexagonal layer architecture. The JavaScript callback mechanism and event-based Node.js project architecture are the foundations of the Node.js project structure processing model.
Let’s now examine each component of the clean reference architecture and the development process by which a web server is built with Node.js callback functions.
Parts of the Node.js architecture:
- Request:
Depending on the expensive tasks a user wants to complete in a web application, incoming requests can be blocked (complicated) or non-blocking (simple).
- Node.js server:
A server-side platform called Node.js server accepts user and development team process requests and then returns results to the corresponding users.
- Event queue:
A node’s event queue. Incoming client requests are stored by the js server and sent one at a development time into the Event Loop.
- Thread pool:
All the threads available for doing certain boring and error-prone tasks necessary to satisfy client requests are collected in a thread pool.
- Event loop:
Event Loop continuously accepts requests, handles them, and sends appropriate responses to external customer engagement.
- External resources:
For handling client requests that are being blocked, other resources are needed. We may use these resources for processing, data storage, or other purposes.
The workflow of Node.js architecture:
The workflow for a Node.Js project structure-based web server is often similar to the one shown in the diagram below. Let’s examine this operational control flow in greater depth.
To communicate with the web application, clients request the web server. Demands may be blocking or non-blocking:
- Querying for data
- Deleting data
- Updating the data
- The incoming requests are retrieved by the Node.js project structure and added to the Event Queue.
- Following that, each request is routed individually through the Event Loop. It determines whether the requests are straightforward enough not to need outside assistance.
- Simple requests (non-blocking activities), like I/O Polling, are processed by Event Loop, and the results are returned to the appropriate clients.
A single complex request receives a single thread from the internal Thread Pool. These threads perform a specific blocking request using external resources, like the file system, database, and computer.
The responses are sent to the event loop once the action has been completed, and the Event Loop then delivers the response back to the Client.
Node.js basic architecture:
Node.js’s key characteristics are:
- Event-Driven Architecture
- Non-Blocking I/O Model
- Asynchronous by Default
What is Node.js used for:
The goals of Node are to address the following issues:
Expensive I/O operations:
While working or rendering with a Web Request. The following are examples of these events for background tasks:
- Accessing File System
- Access Database for Information
- Accessing APIs
- Accessing System Information
Let’s first analyze the traditional approach:
Waiting for these I/O operations consumes a significant amount of thread processing time. Let’s imagine a situation where a single network request must access data from the database, access information from files, and seek information specific to a particular system before responding.
In the following scenario, each request would be handled by a single thread. Access to a database-dependent service, a file system, and network information are all included in this request. The series to execution process is as follows:
- The thread makes a query in the database, which takes 500 milliseconds for the development process. While this happens, the main line waits for the database request to be handled.
- Once the database request has been handled, the thread will contact the file system, which is a time-consuming I/O procedure. Let’s say this process takes 300 milliseconds. The thread will enter waiting mode once more until the I/O request has been processed.
- We need to obtain the system information (let’s say disc space) after the I/O request has finished. This request requires access to the OS data, which takes some time to fetch. The main thread is once more waiting for the retrieval of System Information.
The main thread designated for the request is waiting for each expensive I/O request, Database analytics service Query, and OS Information to finish. This model wastes a lot of the threads’ processing power because they spend most of their time waiting rather than processing data.
Technical analysis for the same:
It is Expensive to Assign Independent Threads to Each Request. A separate Thread handles each request in languages like C# and Java. The method has a flaw because the server has a fixed number of threads in the thread pool since there is always the same number of threads in the thread pool, the maximum number of requests that we can process at once.
If an expensive I/O operation is required to process a request, the thread must remain active while it waits for the lengthy procedure to be finished. While waiting for I/O operations, much thread processing time is lost. The thread could be idle for a long, wasting time and resources.
Node.js working structure:
Because Node ensures it, these Async Tasks are Event Based and Asynchronous. We must build an event-based access layer architecture to prevent the Main Thread from waiting for the Asynchronous Request to complete. While the expensive I/O request is being processed, the Main Thread can switch to another task. It guarantees that the Main Thread is continuously active. An event is fired, indicating that the time-consuming activity has been completed and that the main thread can continue processing the request.
Node.js is a single-threaded event loop:
Node is frequently mistaken for being multi-threaded. It has a single-threaded event loop reference architecture as its foundation. It uses a single thread for its event loop. On this event thread, all requests have been received. Both asynchronous and synchronous execution is part of every request. The main thread should carry out only the synchronous portion of the request. The Main Thread creates a Background thread to handle asynchronous I/O operations as soon as they occur.
Node is still called single-threaded even though there are several background threads since a single thread handles all requests. Internal management by Node controls how async processes are executed on the background threads.
Node has a non-blocking I/O model:
The main thread of a node does not wait for an externally initiated time-consuming process to finish. The Main Thread does not wait for the I/O single Function to finish execution while the Background Thread is doing it. The Main Thread is free to accept more Requests while the Async complex computation Task is completed in the background. After the Background Thread has finished running, it switches back to the Main Thread to continue running. Node’s Main Thread alternates between various Requests continually to carry out its Synchronous Part.
Event-based, Instead of waiting for I/O operation:
The Background Thread notifies the Main Thread using an Event-Based Approach. Each Async complex computation Task has a Callback Function attached to it, and when it is finished, the Background Thread raises an Event to inform the Main Thread that the Async complex computation Task has been completed. The main thread may be occupied processing another client’s request; in this case, the callback requests are put on hold while it waits for the main thread to become available.
Advantages of Node.js architecture:
When compared to other server-side languages, Node.js project reference Architecture has several features that offer the server-side platform a clear advantage:
- It is quick and simple to manage several concurrent client requests.
The Node.js server offers effective handling of a large number of incoming requests through the usage of Event Queue and limited thread Pool.
- There is no need to create a more limited thread.
There is no need to construct additional limited threads because Event Loop processes each Client’s request individually. Instead, one thread is adequate to manage an incoming blocked request.
- Less memory and resources are needed.
Because it manages incoming client requests, the Node.js server often uses less memory and resources. Since each concurrent request is handled separately, the process uses less memory.
Compared to servers produced using other server development technologies, these benefits help Node. Js-based servers are much faster and more responsive.
Conclusion:
Now that you know how the Node.js layer architecture handles heavy client demands, you might be asking how you can develop the skills required to benefit from its growing popularity.
The good news is that you have many excellent options for studying this fascinating and useful skill set at your speed. With a combination of live, instructor-led instruction, self-paced tutorials, and hands-on Node.js projects, we’ll give you a solid foundation in this well-liked platform so that you can graduate prepared for the workplace. Start now to take control of your future!
FAQs:
Node js’s high scalability is how?
In contrast to conventional methods, js uses a single limited thread to perform non-blocking I/O calls; therefore, accepting concurrent connections will consume fewer resources.
Does Node JS work well for complex applications?
It is appropriate for big business initiatives that event handler intricate calculations and data processing. Node. Js and Java are compared in terms of how long it takes to construct a program and Node. Js is easier to understand than Java, resulting in speedier development while using Node.
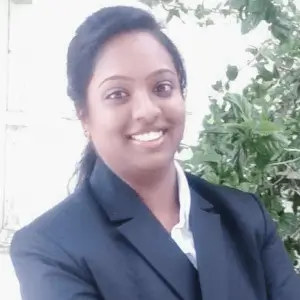
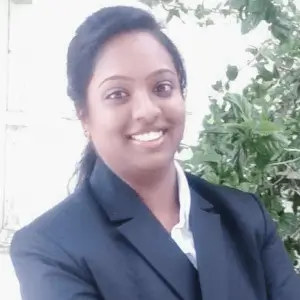
Priyadharshini K.R is a professional blogger with over 3 years of experience who works at Squash Apps. She is dedicated to providing high-quality content to help clients get more visibility on the search engine result pages. She works hard to boost her clients’ online presence through various content writing services. Hailing from Erode, she is passionate about helping people understand content marketing through easily digestible materials.