All of us know Javascript is a “single-threaded non-blocking asynchronous concurrent language, It has a call stack, an event loop, a callback queue, some other apis and stuffs” . We have also heard about V8 – Chrome’s Javascript runtime engine.
Many of us have known already about Javascript’s Event loop, but for the newbies this must be a good read.
Putting the big picture,
Let’s see what happens when this piece of code is executed,
console.log('hi');
setTimeout(function cb() {
console.log('JSC');
},5000);
console.log('how are you');
The result in the console would be,
hi
how are you
JSC
How this code is manipulated in V8 is,
- When that piece of code is executed, console.log(‘hi’) is pushed onto the stack and “hi” is printed in the console.
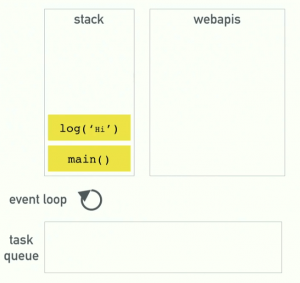
- The next thing is, the timeout function is triggered. The timeout function is not in the stack so a timer is kicked off in the webapis which will run for 5 secs.
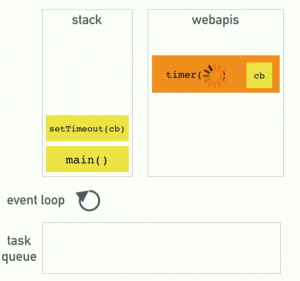
- Since the timer is run by the webapi and also the stack is empty, console.log(‘JSC’) is pushed onto the stack and “JSC” is printed in the console.
- After 5 sec, once the timer is done the function is queued up in the task queue
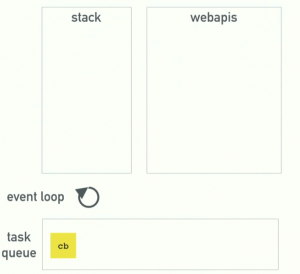
- Here is where the event loop comes into picture, the job of the event loop is to execute the task queue in a FIFO manner only when the stack is empty.
for(var i=0; i<1000000000; i++) {
console.log(i);
if(i===0){
setTimeout(function cd() {
console.log('hi');
},2000);
}
}
I was asked the same question by my CEO one day in my office, the next moment i replied
0,1,2,3 and so on and after 2 secs some where in between
‘hi’ and then all the way to
…. 1000000000
But that’s not right, I totally forgot about the event loop. The exact output would be
0,1,2,3, … ….. …… , 1000000000
hi
You may ask me, what if I give a timeout(function(), 0); , even then the timeout function is going to go to the task queue and it should wait until the event loop is triggered and will be triggered when the stack is empty 😉
“Go through this wonderful presentation by Philip Roberts which was my inspiration”.
Beware of the Event loop!
Happy coding!