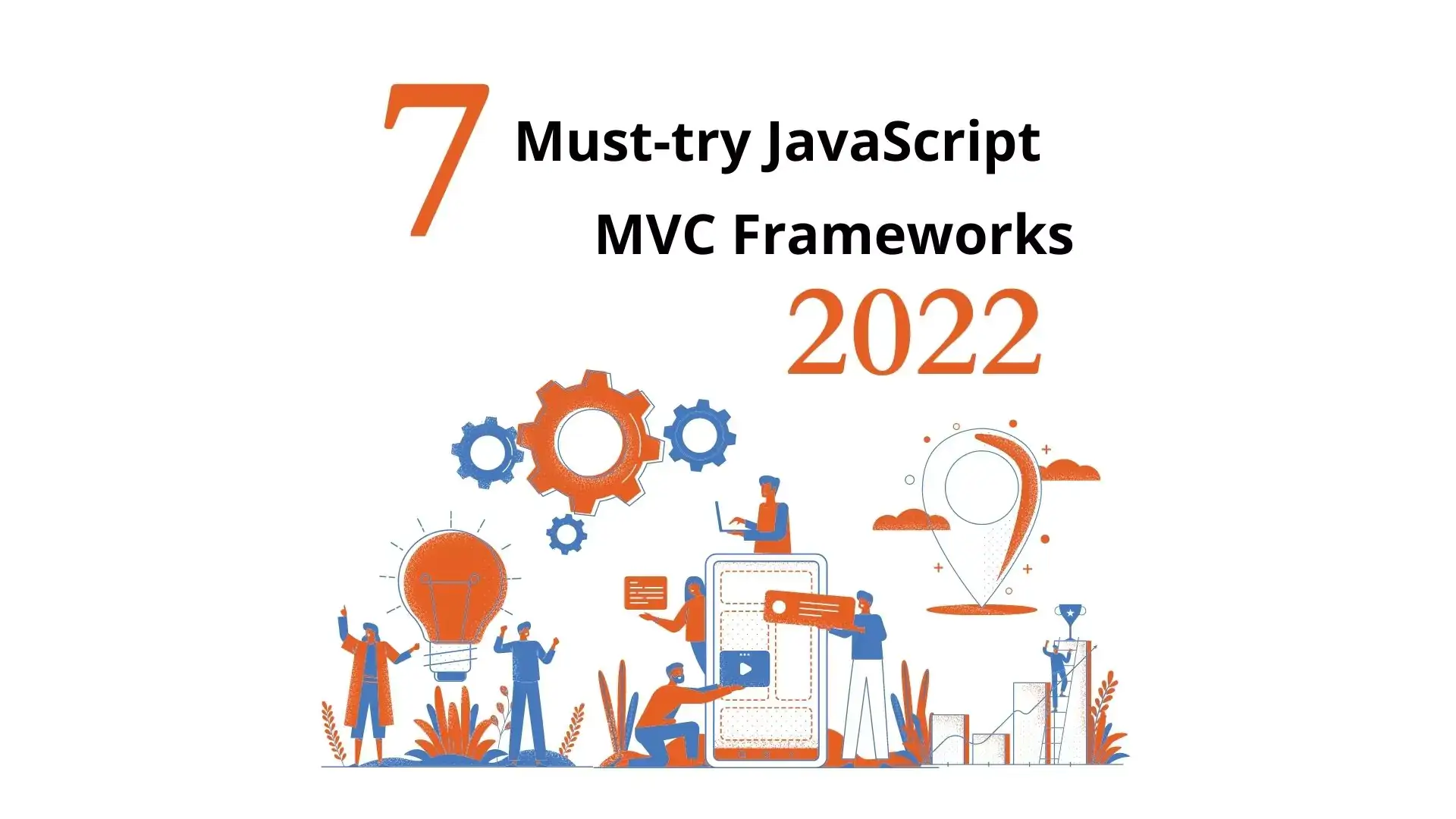
Web development entailed submitting static HTML, CSS, and JavaScript files to an HTTP server.
As software as a service (SaaS) becomes more popular, programs previously only available on the desktop are moved to the browser. JavaScript front-end codebases are becoming larger and more complex to manage as more logic is run in the browser.
As a result, new MVC frameworks that provide structure and guidance when creating front-end codebases for web applications have evolved.
MVC frameworks have become popular among developers because they offer better productivity and maintainable code.
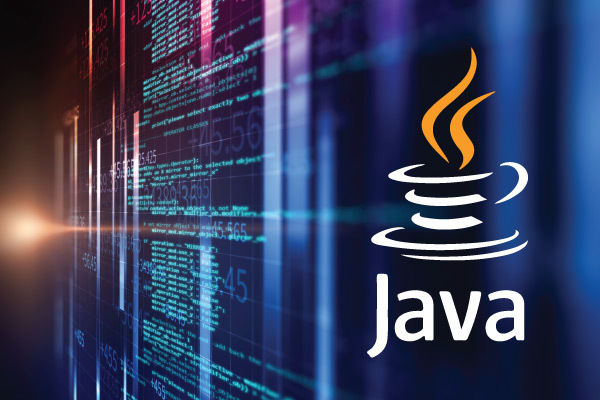
What are MVC Frameworks?
JavaScriptMVC is an open-source framework for developing dynamic Internet applications using jQuery and OpenAjax. MVC frameworks are used with JavaScript to add an abstraction layer to the core language.
It adds a model–view–controller architecture and testing and deployment tools to those frameworks.
How does the MVC Framework work?
An MVC pattern separates input, processing, and output for an application. There are three interconnected parts: the Model, the view, and the controller. Their purpose is to assist in structuring the codebase and divide an application’s concerns into three parts:
Model – Represents the application’s data. The Model corresponds to the type of data a web application handles, such as a user, video, image, or comment. Any subscribed parties within the application are notified when the Model is changed.
View – The application’s user interface is referred to as the view. Views are typically treated as thin adaptors that sit on top of the DOM in most frameworks. The view keeps track of a model and updates itself whenever it changes.
Controller – The controller handles any input, including clicks or browser events. The controller’s responsibility is to update the Model as needed (i.e., if a user changes their name).
All frameworks do not follow the MVC pattern. MVVM and MVP are variations of the MVC pattern used by some frameworks. Read JavaScript Design Patterns if you’re unfamiliar with the MVC pattern or the modifications utilized by various frameworks.
An overview of the most widely used frameworks
This article attempts to compare popular JavaScript frameworks for front-end development in 2021. Becoming an expert at one or more of these JS frameworks will drastically enhance your productivity and help you master programming languages.
The number of functionality supplied by each framework is similar, but their opinions and implementations differ on the best strategy to follow while developing a web application. Some frameworks are fairly flexible in terms of using them, while others demand that you stick to their predefined norms.
By comparing the philosophies of various frameworks, you may select one suitable for your application’s requirements. Each framework has much real-world experience and comes with documentation and community assistance.
Let’s get started!

Backbone.js provides a plugin and add-on library that can provide whatever functionality your application demands.
Backbone is more of a utility library than a full-fledged MVC framework when used without plugins.
Because of Backbone’s modularity, you may fine-tune it to utilize a different templating engine if your application requires it. As a result, Backbone is a good choice for designing a web application with many moving parts.
Pros: unopinionated, great track record of being used in large web apps (WordPress, Rdio, Hulu), source code extremely simple to understand, the gentle learning curve
Cons: External dependencies are required (underscore), memory management can be difficult for novices, there is no built-in two-way binding, it is unopinionated, and it requires plugins to become as feature-complete as other MVC frameworks.
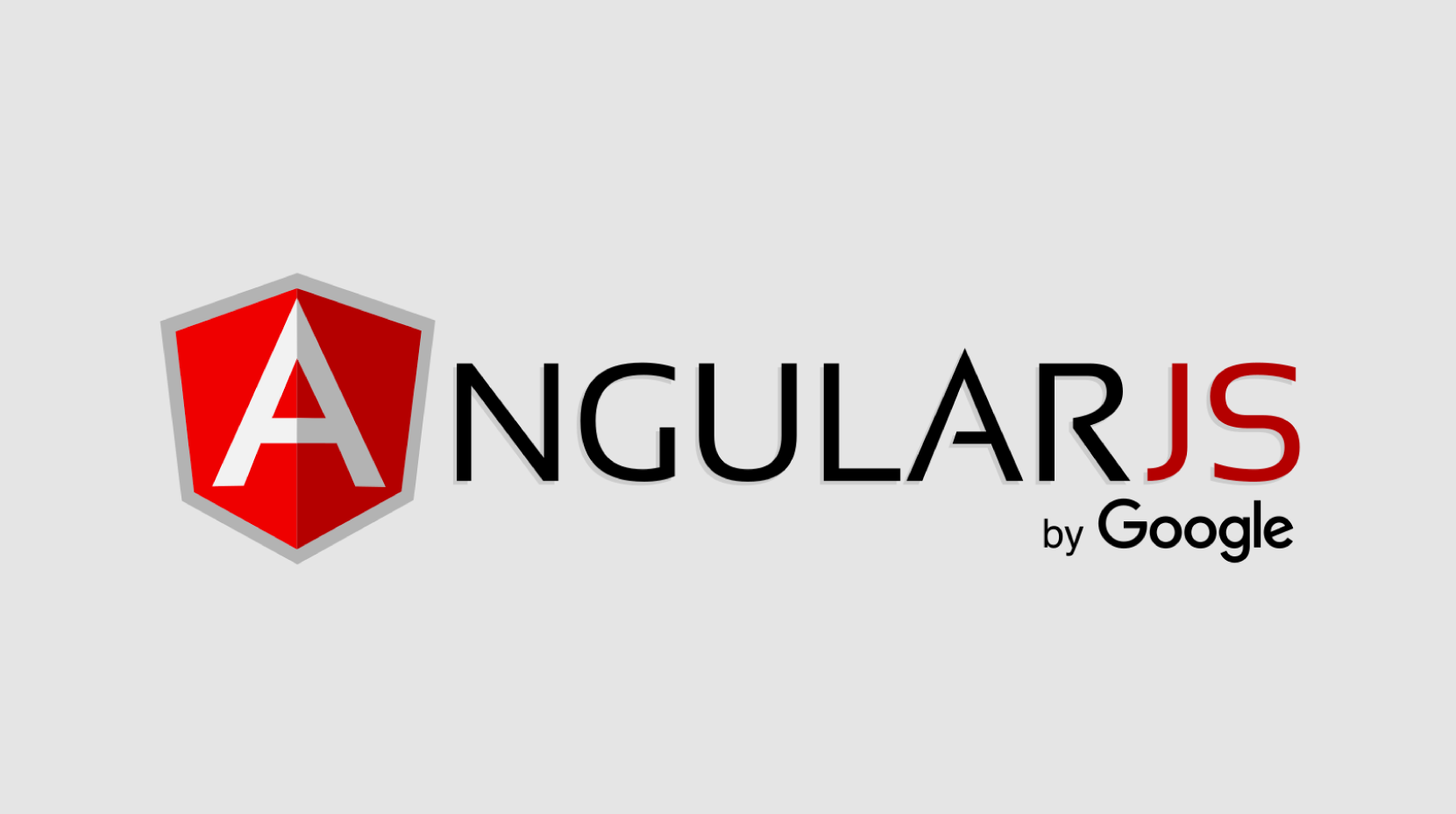
Google created and developed Angular.js, which is rapidly gaining popularity. Using custom HTML tags and components to express the objectives of your application is Angular’s standout feature.
It comes with an HTML generator that lets users construct their domain-specific language; this can be a very useful tool. The approach differs from other frameworks, which attempt to address HTML’s problems by abstracting HTML, CSS, and JavaScript and giving alternate methods for manipulating the DOM.
Pros: Google-backed dependency injection, built-in testing framework, built-in form validation, directives, and extremely easy debugging
Cons: High learning curve, data-binding can be difficult for pages with much data, and transitions between showing and hiding views are difficult to construct.
EmberJS is an opinionated, modular framework that may be straightforward and intuitive to work with if you follow the Ember style of building applications.
Compared to other frameworks, its convention over configuration approach implies it provides an appropriate starting point for the design.
When working with EmberJS, it will seem very familiar if you’ve worked with Ruby on Rails.
Pros: Ember Data makes syncing with JSON APIs much easier, requires little configuration, and follows a convention over the configuration approach.
Cons: Lacks comprehensive testing tools, makes it difficult to incorporate third-party libraries due to its opinionated approach, has a steep learning curve at first, and has an unreliable API.
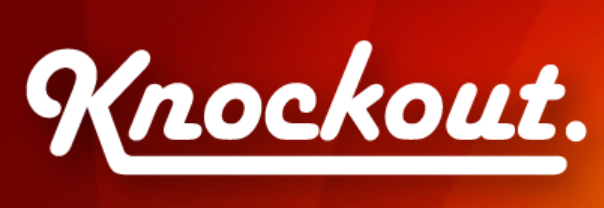
KnockoutJS simplifies dynamic UIs with an MVVM (Model – View – ViewModel) pattern. It provides declarative bindings that build even the most complex UIs while maintaining the underlying data model.
Bindings make custom behaviors like sorting a table simple to create in only minimum lines of code.
As for compatibility, KnockoutJS works with all modern browsers and legacy browsers (Internet Explorer 6).
Pros: Data binding is intuitive and quick to learn, custom events allow for easy implementation of custom behavior, and inter-application connections are managed with a dependency graph, ensuring high performance even for data-heavy applications.
Cons: HTML templates/views can get messy if lots of bindings are used, end-to-end functionality (URL routing, data-access) is not available out of the box, and no third-party dependencies
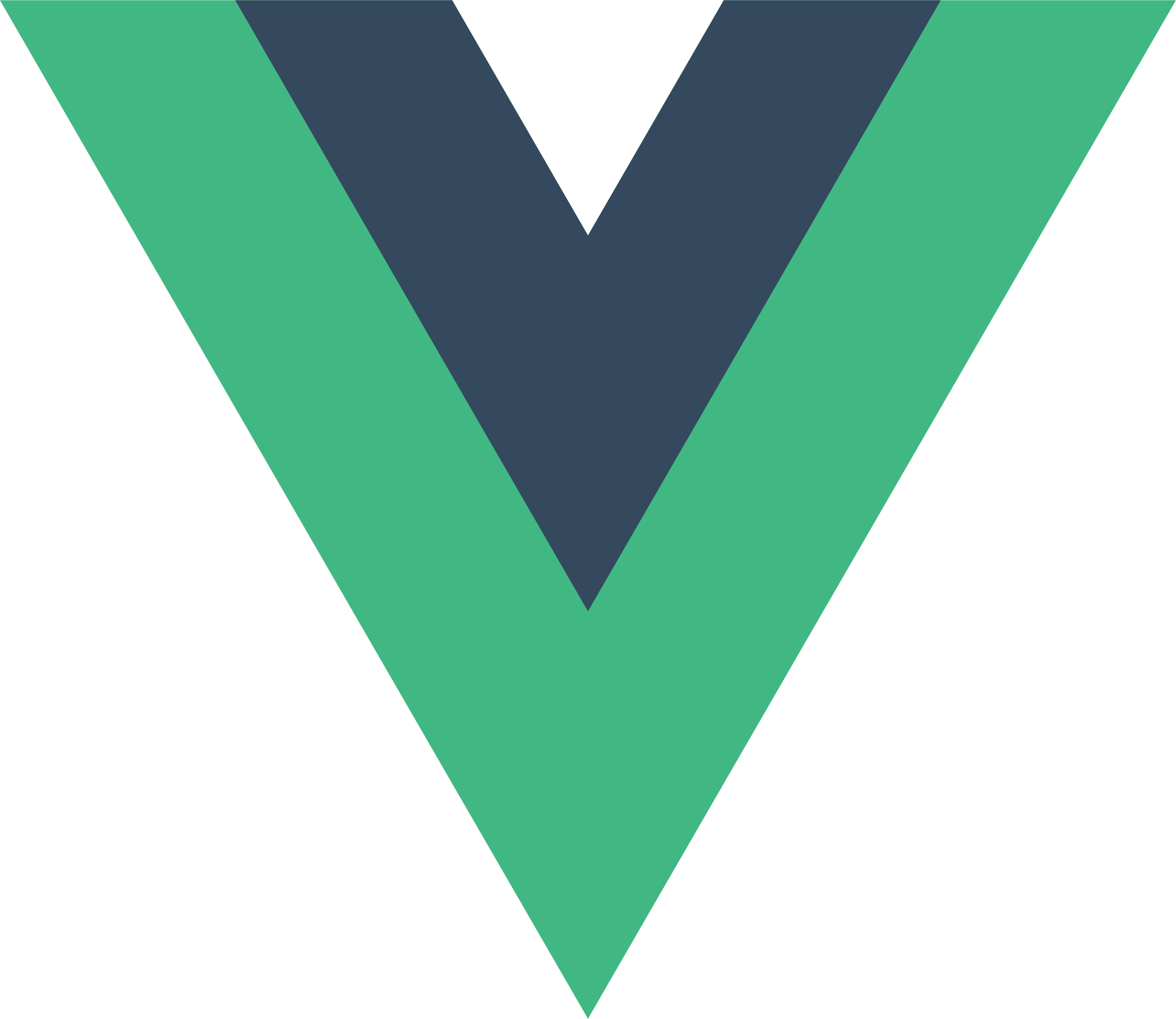
Evan You created Vue.js, a progressive JavaScript framework launched in 2014. Vue is also one of the most rapidly expanding JavaScript frameworks in popularity and acceptance.
Vue.js supports two-way data binding, server-side rendering, and tools with vue-cli. It has extensive online documentation and many free Vue.js tutorials from the community. Vue is also suitable for large-scale projects.
React
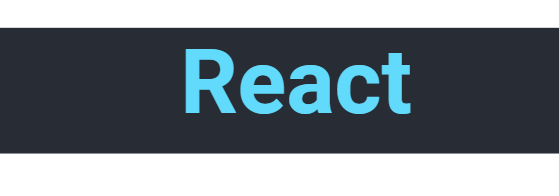
React, created by Facebook and published in 2013, acquired a lot of traction and quickly became the most popular JavaScript framework. React drives Facebook and Instagram user interfaces, making it ideal for high-performance enterprise applications.
React promotes encapsulated components that handle their state and deliver a one-way reactive data flow. React Native may power mobile apps, while React is used on the server.
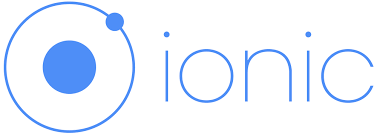
Ionic is a comprehensive open-source SDK for hybrid mobile app development. The first version, launched in 2013, was based on AngularJS and Apache Cordova. It enables programmers to create once and run anywhere.
Ionic allows web developers to use react js, angular, or vuejs to create mobile apps.
Other JS Frameworks worth mentioning
Agility.js
It is a client-side MVC library for Javascript that allows you to write maintainable code without sacrificing development speed.
CanJS
It is a suite of client-side JavaScript architectural frameworks that aim to strike a balance between innovation and stability. It is aimed at experienced developers working on sophisticated projects with a bright future. CanJS is a Javascript MVC Frameworks category tech stack tool. CanJS is an open-source project with 1.9K stars on GitHub and 420 forks.
Spine.JS
The spine is a lightweight and basic framework that doesn’t have a lot of complicated widgets to configure and customize.
It attempts to offer the most user-friendly documentation of any JavaScript framework.
Which one should you pick?
Before choosing a framework for your web application, you should do your homework. You can use the framework you choose to develop complex or unusual features and maintain the app for years to come.
Here are some summaries to aid your decision-making process on which ones to attempt.
Backbone.js should be used if:
- Your web application has many moving parts and requires a lot of flexibility.
- You must be able to easily update or remove components of your web application (i.e., templating engine).
- You’d want to start with a simple solution to understand MVC frameworks better.
Use Angular.js if you are:
- To ensure durability and stability, choose a large, known firm to support your MVC framework. 2. Using a domain-specific language to decrease the complexity of a web application could be beneficial.
- To thoroughly test a functional web application. To test against the MVC framework, you will need a testing framework built from the ground up.
EmberJS should be used if:
- You’ve worked with Ruby before and enjoyed its convention-over-configuration approach.
- You’ll need a framework to enable you to design solutions and prototypes quickly.
- A framework is necessary for your web application to interface with a JSON API with minimal effort.
Use KnockoutJS if:
- Knockout’s MVVM pattern is much better suited to your application structure.
- Frameworks and applications must support legacy browsers such as IE6.
- Your application has a complex dynamic UI, so the framework should create these as cleanly as possible.
All the seven JavaScript frameworks described above are quite popular and most frequently used by web developers worldwide. You’ll have to consider several things to choose the best JavaScript framework.
For example, if you want a framework with strong commercial backing, React or Angular are excellent choices; yet, if you want a framework with a simple learning curve and a large community, Vue.js may be the right pick.
Why are they needed?
Building web pages utilizing a DOM manipulation toolkit like jQuery and utility libraries (underscore, modernizr) can be a lot easier. However, these frameworks lose their utility when utilized to create online applications.
Web applications differ from regular web pages because they require real-time communication with a backend server and greater user engagement. If you handled this behavior without an MVC framework, you’d write messy, unstructured, unmaintainable, and untestable code.
The Benefits of Using the MVC Framework
1. Rapid and Parallel Development:
MVC allows for rapid and parallel development. If an MVC model is used to design a web application, one programmer may work on the view while the other works on the controller to generate the website’s business logic.
As a result, an application built using the MVC model can be completed three times faster than an application built using alternative development patterns.
2. Capacity to Provide Multiple Perspectives:
You can construct numerous views for a model in the MVC Pattern. There is a growing desire for additional ways to access your application today, and MVC development is a wonderful way to meet that demand.
Furthermore, code duplication is minimal because the data and business logic are separated from the display.
3. Asynchronous Technique Support:
The MVC design can also be used with the JavaScript Framework. Now MVC apps can operate with PDF files, site-specific browsers, and desktop widgets.
MVC also offers an asynchronous method, which aids developers in creating a fast-loading application.
4. The Change Does Not Affect The Entire Model:
The user interface changes more frequently in any online application than in the.net development company’s business principles. You make frequent modifications to your online application, such as changing colors, fonts, and screen layouts and introducing new smartphone and tablet device compatibility, which is understandable.
Adding a new view is also simple using the MVC paradigm because the Model component is independent of the Views portion. As a result, any modifications to the Model will not impact the overall design.
5. MVC Model Returns Data Without Formatting:
The MVC pattern returns data with no formatting applied. As a result, the same components can be utilized with any interface and called for use. HTML, for example, may format any data, but it can also be produced with Macromedia Flash or Dreamweaver.
Conclusion
The preceding discussion demonstrates that no “one-size-fits-all” paradigm is appropriate for all cases. Each framework has benefits that make it suited for various situations. To gain a sense of each framework, you should try it out for a short time.
Finally, construct several proof-of-concept prototypes in each framework and compare the results. If you are someone looking for an effective solution to take care of your web-building activities and would like to hire the expertise of a professional JS developer, reach out to us anytime.
Abhinav Sathyamurthy is a professional blogger with over six years of experience covering technical topics such as blockchain, ERP, AI, and other matters.